Function Send-SMS { <# .SYNOPSIS This is a PowerShell script that will send SMS messages from numbers.csv file .DESCRIPTION The script will send SMS message to all the users from numbers.csv file. the csv file contains the name of the user and the mobile number. The headers are Name and Number. The csv file must be stored in C:\Script location and must contain format 44XXXXXXXXXXX. .EXAMPLE This command will send SMS to everyone based on the body Send-SMS -Body " This is SMS message. Regards" .EXAMPLE This command will send SMS to everyone based on the body and your name Send-SMS -Body " This is SMS message. Regards" -Name "John" #> [CmdletBinding()] param ( [Parameter(Mandatory = 'True', HelpMessage = 'Write a SMS message of the problem')] [STRING]$Body, [Parameter(HelpMessage = 'Type your name as the Receiver can verify who the sender is')] [STRING]$Name ) # Pull in Twilio account info $sid = "VWRZf2LEgC8B6XmegD4mKwc3PTCgU7d3" $token = "R4qA7AYdzjRWsR3GfWmYzZ6mfWBt98Fm" $number = "447365541166" $toNumbers = Import-csv "C:\Script\Numbers.csv" #Pull name and number from csv file to send SMS to $url = "https://api.twilio.com/2010-04-01/Accounts/$sid/Messages.json" foreach($toNumbers in $num) { $params = @{ To = $num.Number; From = $number; Body = "$Body. Regards $Name" } #Hash table $p = ConvertTo-SecureString $token -asPlainText -Force $credential = New-Object System.Management.Automation.PSCredential($sid, $p) Invoke-WebRequest $url -Method Post -Credential $credential -Body $params -UseBasicParsing | ConvertFrom-Json | Select-Object sid, body } }
The above will send SMS messages to all members listed from csv file called Numbers. This script logs in to Twilio account and retrieves the number where SMS is sending from and sends the message according to the body. I have broken down each part to explain further.
The below section is where I named the function called Send-SMS. I trigger the script by typing Send-SMS like any other cmdlets. Below the function name, contains help information explaining what the script does and how to execute the command. You will see this help information when typing get-help Send-SMS
Function Send-SMS { <# .SYNOPSIS This is a PowerShell script that will send SMS messages from numbers.csv file .DESCRIPTION The script will send SMS message to all the users from numbers.csv file. the csv file contains the name of the user and the mobile number. The headers are Name and Number. The csv file must be stored in C:\Script location and must contain format 44XXXXXXXXXXX. .EXAMPLE This command will send SMS to everyone based on the body Send-SMS -Body " This is SMS message. Regards" .EXAMPLE This command will send SMS to everyone based on the body and your name Send-SMS -Body " This is SMS message. Regards" -Name "John" #>
The section below the help are list of parameters to use. I have made the Body mandatory as this will be the body of the message. I have made the name as optional to include your name in the body. I have chosen $Body and $Name as my parameters. The $Body is used for writing SMS message and $Name is used to add your name below the message. I have set the name property as optional to have a choice wither the receiver would like to see your name or not.
[CmdletBinding()] param ( [Parameter(Mandatory = 'True', HelpMessage = 'Write a SMS message of the problem')] [STRING]$Body, [Parameter(HelpMessage = 'Type your name as the Receiver can verify who the sender is')] [STRING]$Name }
The next section of the script connects to Twilio API using the API credentials given when signing up to Twilio. After signing up I was given the SID, which is equivalent to username and Auth Token, which is equivalent to password. I copied SID and paste this in $sid variable and the Auth Token in $token variable.
I then have to setup and verify phone number to send SMS messages. This is also done in Twilio by navigating to Phone Numbers -> Verified caller ID. I have verified my personal number but I can choose another number by purchasing a phone number from buy a number tab. Once the phone number is purchased, you verify the number by going back to Verified caller ID and add the number. There are two fields the number field is the full phone number, which includes the + i.e. +447934693985 and the friendly name is the number without the + i.e. 447934693985. The friendly name of the number is added in $number variable.
After the number is added, I created a csv file called Numbers.csv located in C:\Scripts. The csv file contains Number field and list of mobile numbers. I used import-csv cmdlet to import data in a variable called $toNumbers.
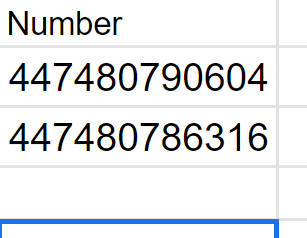
The $url variable contains the web address to connect to Twilio account. # Pull in Twilio account info $sid = "VWRZf2LEgC8B6XmegD4mKwc3PTCgU7d3" $token = "R4qA7AYdzjRWsR3GfWmYzZ6mfWBt98Fm" $number = "447365541166" $toNumbers = Import-csv "C:\Script\Numbers.csv" #Pull name and number from csv file to send SMS to $url = "https://api.twilio.com/2010-04-01/Accounts/$sid/Messages.json"
The next part of the script is the final part of the script that will send SMS. I used ForEach loop to setup the message ready to send. I set the message by creating a hash table called $params. This hash table contains three attributes:
To – This contains the numbers listed in csv file as declared in $toNumbers variable
From – is the number that SMS is sent from. This is declared in $number variable
Body – Is the message to write which is declared in the function parameter. The body also contains the name variable that is also declared in the parameter.
After setting up the hash table. I then created a secured string for the Auth Token by using a variable called $p and then used $credential variable to combine both SID and Auth Token. Once the credential is set, I used invoke-webrequest to connect to Twilio URL with the credentials specified in $credentials and send the SMS based on $param hash table. I used the convertfrom-jason to read message in PowerShell structured way.
foreach($toNumbers in $num) { $params = @{ To = $num.Number; From = $number; Body = "$Body. Regards $Name" } #Hash table $p = ConvertTo-SecureString $token -asPlainText -Force $credential = New-Object System.Management.Automation.PSCredential($sid, $p) Invoke-WebRequest $url -Method Post -Credential $credential -Body $params -UseBasicParsing | ConvertFrom-Json | Select-Object sid, body } }
This is how to execute the script
Send-SMS -body "This is a test message" -name "Test"